Dialogs
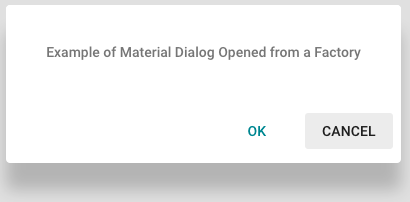
Dialogs are a component of Angular Material.
This repository gives you a layer of abstraction on top of Angular Material's dialog service.
The custom DialogService
allows you to easily open an alert and a confirm using pre-defined defaults that you can modify in angular/services/dialog.service.js
.
export class LandingController() {
constructor(DialogService){
'ngInject';
this.DialogService = DialogService;
this.confirmMessage = '';
}
sampleAlert(){
this.DialogService.alert('This is an alert title', 'You can specify some description text in here.');
}
sampleConfirm(){
this.DialogService.confirm('This is a confirm title', 'Are you sure you want to do delete this file?').then(() => {
this.confirmMessage = 'Success callback';
}, () => {
this.confirmMessage = 'Cancel callback';
}
);
}
}
Custom Dialogs
Another useful feature is the ability to easily call custom dialogs using a folder by feature approach.
Let's start by generating a custom dialog:
php artisan ng:dialog login
This will create a login folder in angular/dialogs
with a sample controller and template.
You can then open this dialog easily using the following sample code:
(function() {
"use strict";
angular.module('app.controllers').controller('LoginController', LoginController);
function LoginController(DialogService) {
var vm = this;
vm.customDialog = customDialog;
var customDialog = function() {
DialogService.fromTemplate('login');
};
});
})();
This example works out of the box.
Elixir, live-reload, DialogService & Artisan generators all work in harmony to achieve this.
Updated less than a minute ago